- Published on
How to configure environment variables for Flutter integration tests in vscode
I've been quite happily writing flutter tests using the vanilla sdk testing modules:
dev_dependencies:
flutter_test:
sdk: flutter
integration_test:
sdk: flutter
But something wasn't working as expected with the environment variables in vscode. Turns out the dart integration with vscode behaves differently to how i've used the debug and launch configurations in vscode. Skip to the end of this post for the solution. I've left my original working out below for future reference.
Test env vars
For some reason the FLUTTER_TEST env var isn't being set on my machine when i expect it, and vscode seems to be using a debug config that i can't influence, so i'm running it on the command line and supplying my own vars e.g.
flutter test integration_test/login_test.dart -d emulator-5554 --dart-define=FLUTTER_TEST=true --dart-define=DEVELOPMENT_MODE=true --dart-define=API_URI=http://192.168.1.246:1337 --dart-define=FIREBASE_EMULATOR_HOST=192.168.1.246
In order to continue using vscode tho, as it's a nice interface. I've added some more logic to the main.dart to turn the logging on and off for flutter test, and the tests themselves supply a bool that indicates they are tests. This completely removes the need to try and set an environment variable via the test runner in vscode.
As a further development, i've split the variables into two types for easier management.
- Application variables - that control the behaviour of the application in production. These are set in firebase remote control
- Development variables - that control things like error reporting and sentry. These are set at runtime in vscode by dart define.
{
"name": "cm_strapi_local",
"request": "launch",
"type": "dart",
"flutterMode": "debug",
"args": [
"--dart-define=DEVELOPMENT_MODE=true", // this enables the firebase emulator settings
"--dart-define=FIREBASE_EMULATOR_HOST=192.168.1.246",
"--dart-define=API_URI=http://192.168.1.246:1337",
"--dart-define=FLUTTER_TEST=true" // this turns off sentry reporting
]
},
Turns out this is a weird way of doing things. You can set code lens to run the tests in a configuration, but i still can't work out how to set the default environment vars! https://dartcode.org/releases/v3-11/
I finally found the answer. Or at least a less unsatisfactory answer. You can add some additional arguments to the startup for every process:
"dart.flutterAdditionalArgs": [
"--dart-define=DEVELOPMENT_MODE=true",
"--dart-define=FLUTTER_TEST=true",
"--dart-define=API_URI=http://192.168.1.246:1337",
"--dart-define=FIREBASE_EMULATOR_HOST=192.168.1.246"
],
Still not exactly what i'm after, but i can now run the entire test suite from within vscode. https://dartcode.org/releases/v3-18/ https://github.com/Dart-Code/Dart-Code/issues/3462 https://dartcode.org/releases/v3-26/
Turns out that this is actually what i was looking for https://dartcode.org/releases/v3-38/. A way to set the env vars for all the tests at once.
#3854: It’s now possible to override the Run/Debug launch configurations used by the Test Runner by specifying a "templateFor" field on a launch.json configuration. The value should be a path prefix for which tests it should apply to (an empty string means it applies to all tests). The noDebug field should be set explicitly if the config should only apply to either Run or Debug, otherwise it will apply to both.
{
"name": "Run/Debug Tests",
"request": "launch",
"type": "dart",
"env": {
// if we need env vars
},
// toolArgs to always be set when running tests
"toolArgs": [
"--dart-define=DEVELOPMENT_MODE=true",
"--dart-define=FLUTTER_TEST=true",
"--dart-define=FIREBASE_EMULATOR_HOST=192.168.1.246",
"--dart-define=API_URI=http://192.168.1.246:1337"
],
"templateFor": ""
},
And now it's running fantastically. Or at least failing succesfully.
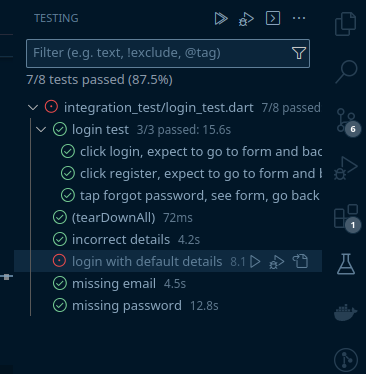